Comprehensive SQL Interview Questions and Answers for Fresher
DBMS and SQL interview question and answer with detailed concept
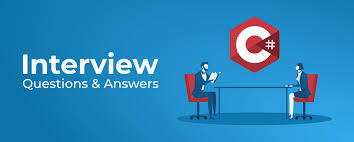
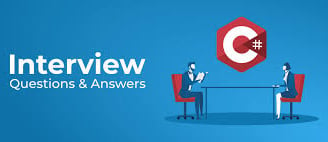
Introduction to SQL and Databases
Structured Query Language, commonly known as SQL, serves as the backbone for database management and operations. It is a powerful tool designed to facilitate communication with databases, allowing users to perform a myriad of functions such as querying data, updating records, and managing database systems. Databases, in essence, are organized collections of data that can be easily accessed, managed, and updated. They are foundational to various applications and services, providing a structured way to store and retrieve large volumes of information.
Databases can be broadly categorized into two types: relational and non-relational. Relational databases, such as MySQL, PostgreSQL, and Oracle, organize data into tables with predefined relationships, making it easier to enforce data integrity and execute complex queries. Non-relational databases, often referred to as NoSQL databases, like MongoDB and Cassandra, store data in a more flexible, schema-less manner, which is particularly useful for handling unstructured data and accommodating rapid scalability.
SQL's significance in the realm of relational databases cannot be overstated. It provides a standardized way to interact with the data, ensuring that users can efficiently perform tasks such as data insertion, update, deletion, and querying. This standardization is critical for maintaining data consistency, integrity, and security across various systems. SQL is employed across diverse industries, from finance and healthcare to e-commerce and technology, underscoring its versatility and indispensability.
For freshers stepping into the world of database management, mastering SQL is a pivotal step. It not only equips them with the necessary skills to manipulate and manage data but also enhances their ability to solve real-world problems effectively. Understanding SQL and its applications prepares candidates to tackle a wide range of interview questions, setting a solid foundation for a successful career in data management and analysis.
Basic SQL Queries and Syntax
Understanding basic SQL queries and syntax is essential for anyone aspiring to work with databases. These foundational elements form the backbone of SQL proficiency and are often the focus of interview questions aimed at freshers. Let's explore some of the fundamental queries and their usage.
The SELECT statement is the cornerstone of SQL queries. It is used to retrieve data from one or more tables. For example:
SELECT * FROM employees;
This query retrieves all columns from the "employees" table. To fetch specific columns, you can do:
SELECT first_name, last_name FROM employees;
The WHERE clause is used to filter records based on specified conditions. For instance:
SELECT * FROM employees WHERE department = 'Sales';
This query fetches all employees who work in the Sales department. Combining multiple conditions with AND or OR operators is also possible:
SELECT * FROM employees WHERE department = 'Sales' AND salary > 50000;
Basic JOIN operations are crucial for combining data from multiple tables. The most common type is the INNER JOIN, which retrieves records with matching values in both tables:
SELECT employees.first_name, departments.department_name FROM employees INNER JOIN departments ON employees.department_id = departments.department_id;
This query joins the "employees" and "departments" tables on the department_id field and returns the first name of employees along with their respective department names.
Simple aggregations like COUNT, SUM, and AVG are used to perform calculations on data sets. For example, to count the number of employees:
SELECT COUNT(*) FROM employees;
To calculate the total salary of all employees:
SELECT SUM(salary) FROM employees;
And to find the average salary:
SELECT AVG(salary) FROM employees;
These basic SQL queries and syntax are indispensable for any SQL-related job interview. A solid grasp of these fundamentals will not only help in cracking interviews but also lay a strong foundation for more advanced SQL concepts.
Advanced SQL Queries
As you progress in your SQL journey, mastering advanced SQL queries becomes essential. These queries go beyond basic SELECT statements to include subqueries, complex JOINs, and aggregate functions, all of which are crucial for sophisticated data analysis.
Subqueries, also known as nested queries, are queries within another SQL query. They can be used in SELECT, INSERT, UPDATE, or DELETE statements. For example, to find employees with salaries higher than the average salary, you can use a subquery:
SELECT employee_id, employee_name FROM employees WHERE salary > (SELECT AVG(salary) FROM employees);
Complex JOINs are another critical aspect of advanced SQL queries. While basic JOINs like INNER JOINs are useful, advanced scenarios often require LEFT JOINs, RIGHT JOINs, or FULL JOINs. For instance, a LEFT JOIN can be used to retrieve all records from the left table and the matched records from the right table:
SELECT employees.employee_id, employees.employee_name, departments.department_name FROM employees LEFT JOIN departments ON employees.department_id = departments.department_id;
Advanced aggregate functions such as SUM(), AVG(), MAX(), and MIN() allow for more detailed data analysis. These functions can be used with the GROUP BY clause to group rows that have the same values in specified columns into summary rows. For example, to find the total sales by each salesperson:
SELECT salesperson_id, SUM(sales_amount) FROM sales GROUP BY salesperson_id;
Window functions provide another layer of analytical capability. Unlike aggregate functions, window functions perform calculations across a set of table rows related to the current row. For example, the ROW_NUMBER() function can assign unique numbers to rows within a partition of a result set:
SELECT employee_id, employee_name, salary, ROW_NUMBER() OVER (PARTITION BY department_id ORDER BY salary DESC) AS rank FROM employees;
Incorporating these advanced SQL techniques into your queries can significantly enhance your ability to perform complex data analysis, making you a more effective and efficient SQL practitioner.
SQL Database Design and Normalization
Database design and normalization are critical components in creating efficient and effective SQL databases. A well-structured database is essential to ensure data integrity, reduce redundancy, and facilitate seamless data retrieval. Poorly designed databases can lead to various issues, such as data anomalies, inconsistencies, and difficulties in maintaining and scaling the database.
Normalization is the process of organizing data within a database to reduce redundancy and improve data integrity. This is achieved by dividing large tables into smaller, more manageable tables and defining the relationships between them. There are several normal forms (NFs), each with specific requirements:
First Normal Form (1NF): A table is in 1NF if all its columns contain atomic (indivisible) values and each column contains only one type of data. For example, a table with columns for student names and their courses should store each course in a separate row, rather than combining multiple courses in a single cell.
Second Normal Form (2NF): A table is in 2NF if it is in 1NF and all non-key attributes are fully functionally dependent on the primary key. This means that each attribute must depend on the entire primary key, not just a part of it. For instance, in a table with a composite key of student ID and course ID, all other columns should depend on both the student ID and course ID, not just one of them.
Third Normal Form (3NF): A table is in 3NF if it is in 2NF and all attributes are only dependent on the primary key. This eliminates transitive dependencies, where a non-key attribute depends on another non-key attribute. For example, if a table has a column for the student's department, the department information should be stored in a separate table, linked to the student table by a foreign key.
Boyce-Codd Normal Form (BCNF): A table is in BCNF if it is in 3NF and every determinant is a candidate key. This form addresses certain anomalies not covered by 3NF. For instance, if a table has multiple candidate keys, BCNF ensures that every candidate key uniquely identifies each row.
While normalization is crucial for database design, there are scenarios where denormalization might be appropriate. Denormalization involves combining tables to reduce the complexity of queries and improve performance. This can be beneficial in read-heavy environments where the overhead of join operations is significant. However, denormalization should be approached with caution, as it can introduce redundancy and data anomalies.
SQL Performance Tuning and Optimization
SQL performance tuning is a critical skill for database administrators and developers alike. Efficiently optimized SQL queries and well-structured databases can lead to significant performance improvements, ensuring faster data retrieval and better application responsiveness. One of the primary techniques for enhancing SQL performance is the use of indexing.
Indexes are special data structures that facilitate quick look-ups of records in a database table. By creating indexes on columns frequently used in WHERE clauses, JOIN conditions, and ORDER BY statements, you can drastically reduce the time it takes to retrieve data. However, it's important to balance indexing, as excessive or unnecessary indexes can lead to increased overhead during data manipulation operations like INSERT, UPDATE, and DELETE.
Another critical aspect of SQL performance tuning involves query optimization strategies. Writing efficient SQL queries by minimizing the use of nested subqueries, avoiding the use of SELECT *, and using appropriate JOIN types can significantly enhance performance. Additionally, rewriting complex queries using Common Table Expressions (CTEs) or temporary tables can sometimes yield better results.
To understand and improve query performance, the use of EXPLAIN plans is indispensable. The EXPLAIN command provides detailed insights into how the SQL engine executes a query, including information about the join order, index usage, and the estimated cost of each operation. By analyzing the EXPLAIN plan, you can identify bottlenecks and optimize your queries accordingly.
Common performance pitfalls include the absence of proper indexing, inefficient query design, and the use of functions on indexed columns within WHERE clauses. To avoid these issues, ensure that your database schema is well-designed, with appropriate normalization and indexing strategies. Regularly monitor and analyze query performance, making adjustments as needed to maintain optimal efficiency.
In essence, mastering SQL performance tuning and optimization techniques, such as proper indexing, efficient query design, and the use of EXPLAIN plans, is essential for maintaining high-performing SQL databases and ensuring that your applications run smoothly and efficiently.
Practical SQL Scenarios and Sample Questions
SQL (Structured Query Language) is a fundamental skill for anyone aiming to work with databases. Freshers often face a variety of SQL questions during interviews, ranging from basic concepts to more complex scenarios. Below is a compilation of practical SQL interview questions and answers designed to provide a comprehensive study guide. These questions cover a broad spectrum of topics including database design, normalization, and performance tuning, ensuring a thorough preparation for SQL interviews.
1. What is SQL?
SQL stands for Structured Query Language. It is used to communicate with databases. According to ANSI (American National Standards Institute), SQL is the standard language for relational database management systems. SQL statements are used to perform tasks such as update data on a database or retrieve data from a database.
2. What is a relational database?
A relational database is a type of database that stores and provides access to data points that are related to one another. Relational databases are based on the relational model, an intuitive, straightforward way of representing data in tables. In a relational database, each row in the table is a record with a unique ID called the key.
3. Explain normalization and its types.
Normalization is the process of organizing the data in the database to reduce redundancy and improve data integrity. There are several normal forms (NF) used to achieve normalization:
First Normal Form (1NF): Ensures that the table is organized into rows and columns, with each column containing atomic values, and each column containing values of a single type.
Second Normal Form (2NF): Builds on 1NF by removing partial dependencies; that is, attributes should depend on the whole primary key.
Third Normal Form (3NF): Ensures that no transitive dependencies exist, meaning non-key attributes should not depend on other non-key attributes.
4. How can you improve SQL query performance?
Improving SQL query performance can be achieved by:
Using indexes to speed up data retrieval.
Avoiding the use of SELECT * and only selecting the columns that are necessary.
Writing efficient joins and subqueries.
Optimizing database schema and normalization.
Using appropriate data types and constraints.
5. What is a JOIN in SQL and what are its types?
A JOIN clause is used to combine rows from two or more tables, based on a related column between them. Types of JOINs include:
INNER JOIN: Returns records that have matching values in both tables.
LEFT JOIN (or LEFT OUTER JOIN): Returns all records from the left table, and the matched records from the right table. The result is NULL from the right side if there is no match.
RIGHT JOIN (or RIGHT OUTER JOIN): Returns all records from the right table, and the matched records from the left table. The result is NULL from the left side when there is no match.
FULL JOIN (or FULL OUTER JOIN): Returns all records when there is a match in either left or right table.
These sample questions and answers provide a foundation for understanding SQL and its applications in real-world scenarios. By mastering these concepts and practicing with practical examples, freshers can significantly enhance their SQL proficiency and confidence during interviews.