.NET and C# Interview Questions and Answers
.NET and C# Interview Questions and Answers for Freshers: A Comprehensive Guide
7/17/202419 min read
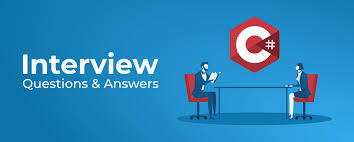
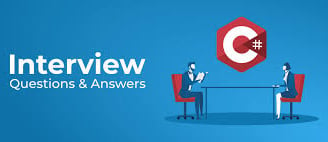
Introduction to .NET and C#: Why It's Important for Freshers
.NET and C# are pivotal technologies in the realm of software development, particularly significant for freshers embarking on their careers in this field. The .NET framework, developed by Microsoft, provides a versatile platform for building a wide array of applications, ranging from web and desktop to mobile solutions. C#, a robust and versatile programming language, seamlessly integrates with the .NET framework, empowering developers to create high-performance, scalable, and secure applications.
The importance of .NET and C# in modern software development cannot be overstated. These technologies are extensively utilized in various sectors, including finance, healthcare, e-commerce, and entertainment. The comprehensive libraries and tools provided by the .NET ecosystem facilitate rapid development, reducing time-to-market for new applications. Moreover, the support for cross-platform development through .NET Core and .NET 5/6 ensures that applications built using C# can run on different operating systems, such as Windows, Linux, and macOS, offering greater flexibility and reach.
For freshers, acquiring proficiency in .NET and C# is a strategic move that can significantly enhance their employability. The demand for developers skilled in these technologies remains high, with numerous organizations seeking talent capable of leveraging the full potential of the .NET framework and C#. Additionally, the strong community support, extensive documentation, and a plethora of learning resources available for .NET and C# make it easier for newcomers to learn and master these technologies.
Understanding .NET and C# not only provides freshers with a competitive edge in the job market but also equips them with the skills necessary to tackle complex software development challenges. As the industry continues to evolve, the relevance and application of these technologies are expected to grow, making them indispensable tools for any aspiring software developer.
Core Concepts of .NET Framework
The .NET framework is an integral platform for building and running applications on Windows. Understanding its core concepts is crucial for freshers entering the field. Let's delve into some fundamental components that form the backbone of the .NET ecosystem.
The Common Language Runtime (CLR) is the execution engine for .NET applications. It handles memory management, security, exception handling, and more. Think of CLR as the heart of the .NET framework; it ensures that applications run smoothly by providing a managed execution environment.
Next, the Common Type System (CTS) defines how types are declared, used, and managed in the runtime. CTS ensures that objects written in different .NET languages can interact with each other. For example, a string in C# is treated the same way as a string in VB.NET, thanks to CTS.
The Common Language Specification (CLS) is a set of rules that language designers follow to ensure interoperability among .NET languages. If a component adheres to the CLS, it can be used across different .NET languages. This promotes language integration and code reusability within the .NET ecosystem.
The .NET Standard Library is a collection of APIs that are common to all .NET implementations. It provides a uniform set of functionalities for building applications, whether they are running on .NET Framework, .NET Core, or Xamarin. By targeting the .NET Standard, developers can create libraries that are compatible across various .NET platforms, enhancing code sharing and reducing duplication.
These components - CLR, CTS, CLS, and the .NET Standard Library - work together harmoniously to form the robust .NET ecosystem. For instance, when a C# application is compiled, the source code is converted into Intermediate Language (IL) code. The CLR then compiles this IL code into machine code specific to the operating system. Throughout this process, CTS ensures type compatibility, and CLS guarantees that the code can be used across different .NET languages. The .NET Standard Library provides the essential APIs required for the application to run, making the entire ecosystem cohesive and efficient.
Fundamental C# Programming Concepts
Understanding fundamental C# programming concepts is crucial for any fresher preparing for .NET and C# interviews. These concepts form the building blocks of problem-solving and application development in C#. Let's delve into some of these key topics.
Variables and Data Types
Variables in C# are used to store data, which can be of different data types such as int, float, double, char, and string. For example:
int age = 25;
float height = 5.9f;
string name = "John";
Choosing the correct data type is essential for efficient memory management and precise computations.
Operators
C# supports various operators such as arithmetic (+, -, *, /), relational (==, !=, <, >), and logical operators (&&, ||, !). These operators are used to perform operations on variables and values. For instance:
int result = 5 + 3;
bool isEqual = (5 == 3);
Control Structures
Control structures such as if-else and switch-case allow you to execute code conditionally. For example:
if (age > 18)
{
Console.WriteLine("Adult");
}
else
{
Console.WriteLine("Minor");
}
switch (day)
{
case 1:
Console.WriteLine("Monday");
break;
case 2:
Console.WriteLine("Tuesday");
break;
}
Loops
Loops are used to execute a block of code repeatedly. Common loops in C# include for, while, and do-while. For example:
for (int i = 0; i < 5; i++)
{
Console.WriteLine(i);
}
while (i < 5)
{
Console.WriteLine(i);
i++;
}
Exception Handling
Exception handling is crucial for building robust applications. C# provides try-catch blocks to handle exceptions gracefully:
try
{
int divisor = 0;
int result = 10 / divisor;
}
catch (DivideByZeroException ex)
{
Console.WriteLine("Cannot divide by zero.");
}
Mastering these fundamental concepts in C# will equip you with the essential skills required to tackle interview questions effectively and develop reliable applications.
Object-Oriented Programming (OOP) in C#
Object-Oriented Programming (OOP) is a paradigm that uses "objects" to design software. In C#, OOP principles are foundational and essential for developing robust and scalable applications. The key concepts of OOP include classes, objects, inheritance, polymorphism, encapsulation, and abstraction.
A class in C# acts as a blueprint for creating objects. It encapsulates data and behavior through fields (variables) and methods (functions). For example:
public class Car {
public string Make;
public string Model;
public void Drive() {
Console.WriteLine("Car is driving");
}
}
An object is an instance of a class. Using the Car class, you can create an object like so:
Car myCar = new Car();
myCar.Make = "Toyota";
myCar.Model = "Corolla";
myCar.Drive();
Inheritance allows one class to inherit the properties and methods of another, promoting code reusability. For instance:
public class ElectricCar : Car {
public int BatteryCapacity;
}
Polymorphism enables methods to do different things based on the object it is acting upon. This can be achieved through method overriding:
public class Car {
public virtual void Drive() {
Console.WriteLine("Car is driving");
}
}
public class ElectricCar : Car {
public override void Drive() {
Console.WriteLine("Electric car is driving silently");
}
}
Encapsulation is about restricting access to certain components. This is achieved using access modifiers like private, protected, and public:
public class Car {
private string Make;
private string Model;
public void SetMake(string make) {
Make = make;
}
public string GetMake() {
return Make;
}
}
Abstraction simplifies complex systems by modeling classes appropriate to the problem. It hides the complex reality while exposing only the necessary parts:
public abstract class Vehicle {
public abstract void Drive();
}
public class Car : Vehicle {
public override void Drive() {
Console.WriteLine("Car is driving");
}
}
Common interview questions related to OOP in C# might include: "Explain the four pillars of OOP," "What is the difference between an abstract class and an interface?" and "How does C# implement polymorphism?" Freshers can effectively answer these by providing clear definitions, examples, and demonstrating an understanding of how these principles are applied in real-world scenarios.
Common .NET and C# Interview Questions for Freshers
When interviewing for a .NET and C# developer position, freshers can expect to encounter a variety of questions that test their understanding of both theoretical concepts and practical coding skills. Here are some common questions and tips on how to approach them:
1. What is the Common Language Runtime (CLR)?
Interviewers ask this question to gauge your understanding of the .NET framework's core component. The CLR is the virtual machine component of Microsoft's .NET framework, managing the execution of .NET programs. A good answer should highlight its roles, such as memory management, security, and exception handling.
2. Explain the concept of Garbage Collection in .NET.
This question tests your knowledge of memory management in .NET. Garbage Collection automatically releases memory occupied by objects that are no longer in use. Emphasize the benefits, like preventing memory leaks, and describe the different generations in the garbage collector.
3. What is the difference between a class and an object in C#?
This basic question assesses your understanding of Object-Oriented Programming (OOP). A class is a blueprint for creating objects, defining properties and methods. An object is an instance of a class. Provide examples to illustrate the relationship between them.
4. How does exception handling work in C#?
Interviewers use this question to evaluate your ability to write robust code. Discuss the try-catch-finally construct and the importance of handling exceptions to maintain program stability. Mention specific exceptions like System.Exception and custom exceptions.
5. Describe the use of LINQ in C#.
LINQ (Language Integrated Query) allows querying data from different data sources in a concise and readable manner. Explain how it integrates query capabilities directly into the C# language, and provide examples of LINQ queries on collections.
6. What are delegates in C#?
This question checks your understanding of advanced C# features. Delegates are type-safe function pointers, allowing methods to be passed as parameters. Discuss their role in event handling and callback methods, along with a simple example.
7. Can you explain the concept of inheritance in C#?
Inheritance is a fundamental OOP concept. It allows a class (derived class) to inherit fields and methods from another class (base class). Highlight the benefits, such as code reusability and the ability to create a hierarchical relationship between classes.
8. How do you implement a basic data structure, like a linked list, in C#?
This practical question tests your coding skills. Describe the structure of a linked list, including nodes and pointers. Provide a simple implementation, demonstrating how to add, remove, and traverse elements in the list.
Tips for Acing Your .NET and C# Interview
Preparing for a .NET and C# interview can be a daunting task for freshers. However, with the right strategies, you can significantly enhance your chances of success. One of the most important steps is to practice coding problems regularly. Utilize platforms like LeetCode, HackerRank, and CodeSignal to familiarize yourself with common patterns and algorithms. This not only sharpens your coding skills but also builds your confidence.
Understanding the job description is crucial. Carefully read the requirements to identify the specific skills and experiences the employer is looking for. Tailor your preparation to align with these needs, focusing on the core .NET and C# concepts that are most relevant to the role. Additionally, research the company’s tech stack. Knowing the technologies and tools they use will help you prepare relevant examples and show your enthusiasm for the position.
Be ready to discuss your past projects or coursework. Highlight experiences that are pertinent to the job, emphasizing your problem-solving skills and your ability to work with .NET and C#. Discussing your projects not only demonstrates your technical skills but also shows your ability to apply theoretical knowledge in practical scenarios. Prepare a few key points about each project, including the challenges faced and how you overcame them.
Staying calm and confident during the interview is imperative. Anxiety can impede your ability to think clearly and communicate effectively. Practice mock interviews with friends or use online platforms to simulate the interview environment. This practice can help you become more comfortable with the process and reduce nervousness.
Effective communication is another critical aspect. Clearly articulate your thought process when solving problems during the interview. This not only shows your technical knowledge but also your reasoning and problem-solving approach. Listen carefully to the questions, and don't be afraid to ask for clarification if needed. Remember, the interview is as much about assessing your fit for the role as it is about showcasing your skills.
Important Question with Answer
Certainly! Here's a comprehensive list of 100 .NET and C# interview questions and answers:
### Basics of .NET and C#
1. What is .NET Framework?
- .NET Framework is a software development framework developed by Microsoft that includes a large class library called Framework Class Library (FCL) and provides language interoperability across several programming languages.
2. What is C#?
- C# (pronounced as C sharp) is a modern, object-oriented programming language developed by Microsoft. It is designed for building a wide range of applications that run on the .NET Framework.
3. What is CLR (Common Language Runtime)?
- CLR is the runtime environment provided by .NET Framework that manages the execution of .NET programs. It provides services such as memory management, exception handling, and garbage collection.
4. What are the key features of C#?
- Key features include type safety, automatic garbage collection, properties and events, delegates and events, versioning support, and interoperability with other languages.
5. What are the different types of .NET Framework?
- .NET Framework, .NET Core, and .NET 5 (which unifies .NET Core and .NET Framework) are the major types. .NET Core is cross-platform and supports microservices-based architecture.
6. What are the benefits of using C#?
- Benefits include simplicity, scalability, type safety, interoperability, and extensive framework support.
7. What is the difference between .NET Core and .NET Framework?
- .NET Core is cross-platform and supports microservices-based architecture, while .NET Framework is primarily for Windows and has a larger set of libraries.
8. Explain Managed Code and Unmanaged Code.
- Managed code is code that runs under the control of CLR and benefits from features like garbage collection, while unmanaged code is native code that does not run under CLR's control and is managed by the operating system.
9. What is JIT (Just-In-Time) compilation?
- JIT compilation is a technique in which the bytecode (Intermediate Language or IL) is compiled into native machine code at runtime by the CLR when the application starts.
10. What is garbage collection?
- Garbage collection is the process by which the CLR automatically manages memory. It identifies and deletes objects that are no longer needed, freeing up memory and preventing memory leaks.
### C# Language Fundamentals
11. What are the basic data types in C#?
- Basic data types include int, float, double, char, bool, string, etc.
12. What is a namespace in C#?
- A namespace is a logical container for a group of related classes and other types. It helps in organizing code and avoiding name conflicts.
13. What is the difference between value types and reference types in C#?
- Value types store data directly, while reference types store a reference (memory address) to the location where the data is stored.
14. What are the access modifiers in C#?
- Access modifiers control the accessibility of classes, methods, and other members. They include public, private, protected, internal, and protected internal.
15. What is the difference between `const` and `readonly` in C#?
- `const` variables are compile-time constants and cannot be changed after initialization. `readonly` variables can only be assigned a value at declaration or in the constructor and can be modified later.
16. What is a constructor in C#?
- A constructor is a special method that is automatically called when an instance of a class is created. It is used to initialize the object's data.
17. What is method overloading?
- Method overloading allows defining multiple methods with the same name but with different parameters in the same class. The compiler determines which method to call based on the parameters passed.
18. What is method overriding?
- Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. It is achieved using the `override` keyword.
19. What are properties in C#?
- Properties are members of a class that provide a flexible mechanism to read, write, or compute the value of a private field. They are accessed like fields but have logic behind their access.
20. What are indexers in C#?
- Indexers allow instances of a class or struct to be indexed just like arrays. They are defined using `this` keyword and are used to provide array-like access to objects.
### Object-Oriented Programming (OOP) in C#
21. What is encapsulation?
- Encapsulation is the concept of bundling data (variables) and methods (functions) that operate on the data into a single unit (class). It provides data hiding and abstraction.
22. What is inheritance?
- Inheritance is the mechanism by which one class acquires the properties (methods and fields) of another class. It supports code reuse and hierarchical classification.
23. What is polymorphism?
- Polymorphism allows objects of different classes to be treated as objects of a common superclass. It includes method overriding and method overloading.
24. What is abstraction?
- Abstraction is the process of hiding the implementation details and showing only the essential features of an object. It is achieved using abstract classes and interfaces in C#.
25. What is an abstract class?
- An abstract class is a class that cannot be instantiated and is used as a base class for other classes. It may contain abstract methods (methods without implementation) that must be implemented by derived classes.
26. What is an interface?
- An interface in C# defines a contract that classes can implement. It contains only method signatures, properties, events, or indexers. A class implements an interface using the `implements` keyword.
27. What is the difference between abstract class and interface?
- An abstract class can have both abstract and non-abstract members, while an interface can only have method signatures, properties, events, or indexers. A class can inherit from only one abstract class but can implement multiple interfaces.
28. What are sealed classes in C#?
- Sealed classes are classes that cannot be inherited. They prevent other classes from deriving from them.
29. What are delegates in C#?
- Delegates are type-safe function pointers that reference methods with a specific signature. They are used for implementing callbacks and event handling.
30. What is a multicast delegate?
- A multicast delegate is a delegate that can hold references to more than one function. It can invoke multiple methods sequentially.
### Exception Handling and Error Handling
31. What is exception handling?
- Exception handling is a mechanism to handle runtime errors (exceptions) gracefully without terminating the program.
32. What are the keywords used in exception handling?
- Keywords include `try`, `catch`, `finally`, and `throw`.
33. How do you handle exceptions in C#?
- Exceptions are handled using `try`, `catch`, and optionally `finally` blocks:
```csharp
try {
// code that may throw an exception
} catch (ExceptionType e) {
// handle the exception
} finally {
// optional: cleanup code
}
```
34. What is the difference between `throw` and `throw ex`?
- `throw` re-throws the current exception without resetting its stack trace, while `throw ex` resets the stack trace of the exception.
35. What is the `finally` block used for in exception handling?
- The `finally` block is used to execute cleanup code that must be run regardless of whether an exception occurred in the `try` block or not.
### Collections and Generics
36. What are collections in C#?
- Collections are classes that store groups of objects or elements. Examples include `List<T>`, `Dictionary<TKey, TValue>`, `Queue<T>`, `Stack<T>`, etc.
37. What is `List<T>` in C#?
- `List<T>` is a generic collection class that stores elements of a specified type `T`. It allows dynamic resizing and provides methods for adding, removing, and manipulating elements.
38. What are generics in C#?
- Generics allow you to write reusable code by defining type parameters that can be used to create classes, methods, interfaces, and delegates.
39. What is the difference between `IEnumerable` and `IEnumerator`?
- `IEnumerable` represents a collection of objects that can be enumerated (iterated over), while `IEnumerator` provides methods for iterating over the collection.
40. What is a lambda expression in C#?
- A lambda expression is an anonymous function that allows you to define a method inline. It simplifies the syntax for defining delegates and writing LINQ query expressions.
### LINQ (Language Integrated Query)
41. What is LINQ?
- LINQ is Language Integrated Query, a set of features introduced in C# that adds query capabilities to the language syntax. It allows querying data from different data sources using a uniform API.
42. What are the benefits of using LINQ?
- Benefits include type safety, compile-time checking, ease of use, and integration with C# language features.
43. What are the standard query operators in LINQ?
- Standard query operators include `Where`, `Select`, `OrderBy`, `GroupBy`, `Join`, `Aggregate`, etc.
44. Give an example of using LINQ to query a collection.
- Example:
```
csharp
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = numbers.Where(n => n % 2 == 0);
```
45. What is deferred execution in LINQ?
- Deferred execution means that LINQ queries are not executed until their results are actually needed. This allows for optimizations and efficient querying.
### Asynchronous Programming
46. What is asynchronous programming in C#?
- Asynchronous programming allows you to execute code asynchronously without blocking the main thread, improving responsiveness and scalability.
47. What are `async` and `await` keywords in C#?
- `async` is used to define an asynchronous method, while `await` is used to asynchronously wait for the completion of an asynchronous operation.
48. What are tasks in C#?
- `Task` is a representation of an asynchronous operation that can return a result or not. It is used extensively in asynchronous programming.
49. What is the difference between `Task.Run()` and `Task.Factory.StartNew()`?
- `Task.Run()` is a simpler and more efficient way to queue work to the thread pool, while `Task.Factory.StartNew()` provides more control over the creation of tasks.
50. How do you handle exceptions in asynchronous methods?
- Exceptions in asynchronous methods are handled using `try-catch` blocks as usual. Exceptions thrown from an `async` method are propagated back when you `await` the task.
### File Handling and IO Operations
51. How do you read from a file in C#?
- Example using `StreamReader`:
```csharp
using (StreamReader sr = new StreamReader("filename.txt")) {
string line;
while ((line = sr.ReadLine()) != null) {
Console.WriteLine(line);
}
}
```
52. How do you write to a file in C#?
- Example using `StreamWriter`:
```csharp
using (StreamWriter sw = new StreamWriter("filename.txt")) {
sw.WriteLine("Hello, World!");
}
```
53. What is the difference between `File.Open` and `File.Create` methods?
- `File.Open` opens an existing file or creates a new one if it doesn't exist, while `File.Create` creates a new file or overwrites an existing file.
54. How do you work with directories in C#?
- Example using `Directory` class:
```csharp
if (!Directory.Exists("path")) {
Directory.CreateDirectory("path");
}
```
### Reflection
55. What is reflection in C#?
- Reflection allows you to inspect and manipulate metadata of types (classes, methods, properties) at runtime. It provides type information at runtime.
56. How do you use reflection to invoke a method?
- Example:
```csharp
Type type = typeof(MyClass);
MethodInfo method = type.GetMethod("MethodName");
object instance = Activator.CreateInstance(type);
method.Invoke(instance, null);
```
57. What is dynamic keyword in C#?
- The `dynamic` keyword allows you to work with objects whose types are not known at compile time. It enables late binding and dynamic typing.
### Serialization and Deserialization
58. What is serialization?
- Serialization is the process of converting an object into a format that can be easily stored, transferred, or persisted. In C#, it is often used to convert objects into XML or JSON format.
59. How do you serialize an object in C#?
- Example using `XmlSerializer`:
```csharp
XmlSerializer serializer = new XmlSerializer(typeof(MyClass));
using (TextWriter writer = new StreamWriter("file.xml")) {
serializer.Serialize(writer, obj);
}
```
60. What is deserialization?
- Deserialization is the process of converting a serialized format (XML, JSON) back into an object.
### ASP.NET and Web Development
61. What is ASP.NET?
- ASP.NET is a web application framework developed by Microsoft for building dynamic web sites, web applications, and web services.
62. What are the different types of ASP.NET?
- ASP.NET Web Forms, ASP.NET MVC (Model-View-Controller), ASP.NET Web API, and ASP.NET Core are the major types.
63. What is the difference between ASP.NET Web Forms and ASP.NET MVC?
- ASP.NET Web Forms uses a Page Controller pattern, while ASP.NET MVC uses a Front Controller pattern (Model-View-Controller). MVC provides more control over HTML and JavaScript.
64. What is MVC (Model-View-Controller)?
- MVC is a design pattern that separates an application into three main components: Model (data), View (user interface), and Controller (handles user input and requests).
65. What is Razor View Engine in ASP.NET?
- Razor is a syntax for creating dynamic web pages with C# or VB.NET in ASP.NET. It allows embedding server-based code into web pages.
### Entity Framework
66. What is Entity Framework (EF)?
- Entity Framework is an object-relational mapping (ORM) framework for .NET that allows developers to work with relational data using domain-specific objects.
67. What are the different approaches to using Entity Framework?
- Approaches include Database-First, Model-First, and Code-First (which is the preferred approach).
68. What is Code-First approach in Entity Framework?
- Code-First approach allows you to define your model using C# classes first, and then EF creates the database based on the classes.
69. How do you query data using Entity Framework?
- Example using LINQ to Entities:
```csharp
using (var context = new MyDbContext()) {
var query = from c in context.Customers
where c.City == "London"
select c;
var customers = query.ToList();
}
```
70. What are migrations in Entity Framework?
- Migrations allow you to manage changes to your database schema over time. They enable you to update the database schema to match changes in your model classes.
### ASP.NET Core
71. What is ASP.NET Core?
- ASP.NET Core is an open-source, cross-platform framework for building modern, cloud-based web applications and services using .NET.
72. What are the key features of ASP.NET Core?
- Key features include cross-platform support, built-in dependency injection, middleware pipeline, unified MVC and Web API frameworks, and improved performance.
73. What is Dependency Injection (DI) in ASP.NET Core?
- DI is a design pattern used to implement Inversion of Control (IoC) in which dependencies are injected into a class rather than the class creating its own dependencies.
74. How do you implement Dependency Injection in ASP.NET Core?
- Example using built-in container:
```csharp
services.AddScoped<IMyService, MyService>();
```
75. What is Middleware in ASP.NET Core?
- Middleware is software components that are assembled into the pipeline to handle requests and responses. Each middleware component chooses whether to pass the request on to the next component.
### Authentication and Authorization
76. What is authentication?
- Authentication is the process of verifying the identity of a user attempting to access a system or application.
77. What is authorization?
- Authorization is the process of determining whether an authenticated user has permission to perform certain actions or access specific resources.
78. How do you implement authentication in ASP.NET Core?
- Example using JWT (JSON Web Tokens):
```csharp
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options => {
options.TokenValidationParameters = new TokenValidationParameters {
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = Configuration["Jwt:Issuer"],
ValidAudience = Configuration["Jwt:Issuer"],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration["Jwt:Key"]))
};
});
```
79. What is CORS (Cross-Origin Resource Sharing) in ASP.NET Core?
- CORS is a security feature that allows restricting resources (e.g., APIs) on a web server to be requested from another domain outside the domain from which the first resource was served.
### Unit Testing
80. What is unit testing?
- Unit testing is a software testing technique where individual units or components of a program are tested in isolation to ensure they work as expected.
81. What are unit testing frameworks available in C#?
- Frameworks include MSTest, NUnit, and xUnit.NET.
82. How do you write unit tests in C# using MSTest?
- Example:
```csharp
[TestClass]
public class MyTests {
[TestMethod]
public void TestMethod1() {
// Arrange
var calculator = new Calculator();
// Act
var result = calculator.Add(2, 3);
// Assert
Assert.AreEqual(5, result);
}
}
```
### Multithreading and Async Programming
83. What is multithreading?
- Multithreading allows concurrent execution of multiple threads in a process. It improves performance and responsiveness.
84. What are threads in C#?
- Threads are the smallest unit of execution within a process. They allow concurrent execution of code.
85. **How do you create and start a new
thread in C#?**
- Example:
```csharp
Thread thread = new Thread(SomeMethod);
thread.Start();
```
86. What are async and await keywords used for in C#?
- `async` is used to define an asynchronous method, while `await` is used to asynchronously wait for the completion of an asynchronous operation without blocking the thread.
87. What is a deadlock in multithreading?
- A deadlock occurs when two or more threads are blocked forever, waiting for each other to release resources that they need to proceed.
88. What are synchronization primitives in C# multithreading?
- Synchronization primitives such as `lock`, `Monitor`, `Mutex`, and `Semaphore` are used to synchronize access to shared resources and prevent race conditions.
### Networking and Web Services
89. What are Web Services?
- Web Services are software systems designed to support interoperable machine-to-machine interaction over a network. They are typically implemented using SOAP or REST protocols.
90. What is RESTful Web API?
- RESTful Web API is an architectural style for building web services that uses HTTP methods (GET, POST, PUT, DELETE) and URLs to perform CRUD (Create, Read, Update, Delete) operations.
91. How do you consume a RESTful API in C#?
- Example using `HttpClient`:
```csharp
using (var client = new HttpClient()) {
var response = await client.GetAsync("https://api.example.com/data");
if (response.IsSuccessStatusCode) {
var data = await response.Content.ReadAsStringAsync();
Console.WriteLine(data);
}
}
```
### Security
92. What is SQL Injection? How do you prevent it in C#?
- SQL Injection is a security vulnerability that occurs when an attacker inserts malicious SQL code into input fields. Prevent it using parameterized queries or ORM frameworks like Entity Framework.
93. What is XSS (Cross-Site Scripting) attack? How do you prevent it in ASP.NET?
- XSS attack occurs when an attacker injects malicious scripts into web pages viewed by other users. Prevent it using encoding HTML input and output using libraries like AntiXSS.
### Performance Optimization
94. How do you optimize performance in C# applications?
- Techniques include minimizing memory allocations, optimizing loops, using asynchronous programming, caching, and profiling using performance tools.
### Deployment and DevOps
95. How do you deploy an ASP.NET Core application?
- Deploy using tools like Visual Studio Publish, Azure DevOps Pipelines, Docker containers, or directly to cloud services like Azure App Service.
96. What is Docker? How do you use Docker with ASP.NET Core?
- Docker is a platform for building, shipping, and running applications in containers. You can create Docker images for ASP.NET Core applications and deploy them to Docker containers.
### Miscellaneous
97. What are the different types of testing in .NET applications?
- Types include unit testing, integration testing, acceptance testing, performance testing, and security testing.
98. What is the Global Assembly Cache (GAC) in .NET?
- GAC is a machine-wide cache of assemblies that allows sharing of assemblies among multiple applications.
99. What is NuGet?
- NuGet is a package manager for .NET that allows you to find, install, and manage packages (libraries and tools) in your projects.
100. What are design patterns? Give examples of some design patterns used in C#.
- Design patterns are reusable solutions to common software design problems. Examples include Singleton, Factory, Observer, Strategy, and Decorator patterns.
These questions cover a wide range of topics and should provide a solid foundation for .NET and C# interviews. Tailor your preparation based on the specific job role and level you are applying for.
TheTechStudioz©2023
Reframe your inbox
Subscribe to our Blog and never miss a story.
We care about your data in our privacy policy.