Core Java Interview
Create a visually appealing Java interview page with 200 questions and answers to help candidates prepare effectively. Stand out with a well-designed platform.
7/14/202415 min read
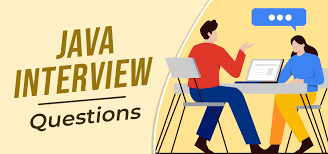
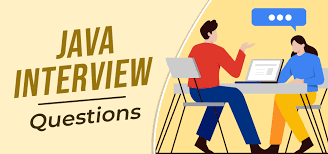
Certainly! Here's a comprehensive list of core Java interview questions for fresher-level positions, along with concise answers. These questions cover fundamental concepts and are designed to help you prepare thoroughly:
Basic Concepts
1. What is Java?
- Java is a high-level programming language developed by Sun Microsystems. It follows the principle of "Write Once, Run Anywhere" (WORA) as it is compiled to bytecode, which can run on any Java Virtual Machine (JVM).
2. What are the key features of Java?
- Features include platform independence, object-oriented programming (OOP) paradigm, strong type checking, automatic memory management (garbage collection), and multithreading support.
3. What is the difference between JDK, JRE, and JVM?
- JDK (Java Development Kit) is a software development kit used for developing Java applications.
- JRE (Java Runtime Environment) is a runtime environment for executing Java applications.
- JVM (Java Virtual Machine) is an abstract machine that provides the runtime environment in which Java bytecode can be executed.
4. Explain the main components of Java.
- The main components are the JVM, which executes Java bytecode; the JDK, which includes tools for developing and debugging; and the JRE, which provides the runtime environment.
5. What is the difference between == and .equals() method in Java?
- `==` is used to compare references (memory addresses) of objects in Java.
- `.equals()` method is used to compare the contents or values of objects.
6. What is the purpose of the `main()` method in Java?
- The `main()` method is the entry point of a Java program. It is where the program starts its execution.
### 2. Variables and Data Types
7. What are the primitive data types in Java?
- `byte`, `short`, `int`, `long`, `float`, `double`, `char`, `boolean`.
8. What is the default value of the elements in an array of integers in Java?
- The default value for numeric types (like integers) in Java is `0`.
9. What are wrapper classes in Java?
- Wrapper classes are classes that allow primitive types to be treated as objects. Examples include `Integer`, `Double`, `Boolean`, etc.
10. What is autoboxing and unboxing in Java?
- Autoboxing is the automatic conversion of primitive types to their corresponding wrapper classes.
- Unboxing is the automatic conversion of wrapper class objects to their corresponding primitive types.
11. What is the difference between `float` and `double` in Java?
- `float` is a single-precision 32-bit IEEE 754 floating point, while `double` is a double-precision 64-bit IEEE 754 floating point.
12. How do you declare constants in Java?
- Constants in Java are typically declared using the `final` keyword. For example:
```java
final int MAX_SIZE = 100;
```
### 3. Control Flow Statements
13. What are the different types of loops in Java?
- `for` loop, `while` loop, `do-while` loop.
14. What is the difference between `break` and `continue` statements?
- `break` statement is used to terminate the loop or switch statement.
- `continue` statement is used to skip the current iteration and continue with the next iteration of the loop.
15. What is the purpose of `switch` statement in Java?
- `switch` statement is used to execute one statement from multiple conditions based on the value of an expression.
16. How do you handle exceptions in Java?
- Exceptions in Java are handled using `try`, `catch`, and `finally` blocks. Code that might throw an exception is enclosed in a `try` block, and exceptions are caught in `catch` blocks.
17. What is the difference between checked and unchecked exceptions?
- Checked exceptions are checked at compile-time, while unchecked exceptions (RuntimeExceptions) are not checked at compile-time.
### 4. Object-Oriented Programming (OOP) Concepts
18. What are the main principles of OOP?
- Encapsulation, Inheritance, Polymorphism, Abstraction.
19. What is inheritance in Java?
- Inheritance is the mechanism where a new class (derived class) is derived from an existing class (base class).
20. What is polymorphism in Java?
- Polymorphism means the ability of an object to take many forms. In Java, it can be achieved through method overriding and method overloading.
21. What is encapsulation in Java?
- Encapsulation is the bundling of data (variables) and methods that operate on the data into a single unit (class). It helps in hiding the data from outside interference and misuse.
22. What is method overriding in Java?
- Method overriding allows a subclass to provide a specific implementation of a method that is already provided by its superclass.
23. What is method overloading in Java?
- Method overloading allows a class to have more than one method having the same name, if their parameter lists are different.
### 5. Classes and Objects
24. What is a class in Java?
- A class is a blueprint or template for creating objects which define its properties and behaviors.
25. What is an object in Java?
- An object is an instance of a class that has its own state (data members) and behavior (methods).
26. How do you create an object in Java?
- An object is created using the `new` keyword followed by a call to a constructor of the class. For example:
```java
MyClass obj = new MyClass();
```
27. What is a constructor in Java?
- A constructor is a special method that is called when an object is instantiated. It initializes the object.
28. Can you have multiple constructors in a class?
- Yes, a class can have multiple constructors. This is known as constructor overloading.
29. What is the purpose of the `this` keyword in Java?
- The `this` keyword refers to the current instance of a class and is used to differentiate between instance variables and local variables when they have the same name.
### 6. String Handling
30. How are strings represented in Java?
- Strings in Java are represented as objects of the `String` class.
31. What is the difference between `String`, `StringBuffer`, and `StringBuilder`?
- `String` objects are immutable (cannot be changed), whereas `StringBuffer` and `StringBuilder` objects are mutable (can be changed).
- `StringBuffer` is synchronized (thread-safe), whereas `StringBuilder` is not synchronized (not thread-safe).
32. How do you concatenate strings in Java?
- Strings can be concatenated using the `+` operator or the `concat()` method.
33. What is string immutability in Java?
- String immutability means that once a `String` object is created, its state cannot be changed. Any modification results in a new `String` object.
34. What is the `StringPool` in Java?
- The `StringPool` is a storage area in the JVM where String literals are stored. Repeated String literals with the same content are not duplicated but are referenced to the same object in the pool.
### 7. Arrays
35. How do you declare an array in Java?
- Arrays are declared using square brackets `[]`. For example:
```java
int[] arr = new int[5];
```
36. What is the difference between an array and an ArrayList?
- An array is a fixed-size data structure whereas an `ArrayList` is a dynamically resizing data structure.
- Arrays can store primitive types and objects whereas `ArrayList` can only store objects.
37. How do you iterate through an array in Java?
- Arrays can be iterated using a `for` loop or an enhanced `for-each` loop. For example:
```java
int[] arr = {1, 2, 3, 4, 5};
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
```
38. How do you find the length of an array in Java?
- The length of an array can be found using the `length` property. For example:
```java
int[] arr = {1, 2, 3, 4, 5};
System.out.println(arr.length); // Outputs: 5
```
### 8. Interfaces and Abstract Classes
39. What is an interface in Java?
- An interface in Java is a reference type, similar to a class, that can contain only constants, method signatures, default methods, static methods, and nested types.
40. Can you instantiate an interface in Java?
- No, you cannot instantiate an interface directly in Java.
41. What is an abstract class in Java?
- An abstract class in Java is a class that cannot be instantiated on its own and is meant to be subclassed. It may contain abstract methods (methods without a body) that subclasses must implement.
42. What is the difference between an interface and an abstract class?
- Interfaces can only have method declarations, constant variables, and default
methods, whereas abstract classes can have method declarations (including abstract methods) as well as concrete methods.
43. When would you use an interface versus an abstract class?
- Use an interface when you want to define a contract for classes to implement, without specifying any implementation details. Use an abstract class when you want to provide a default implementation that subclasses can override if necessary.
44. Can a class implement multiple interfaces in Java?
- Yes, a class can implement multiple interfaces in Java. This is known as multiple inheritance through interfaces.
### 9. Exception Handling
45. What are exceptions in Java?
- Exceptions are events that disrupt the normal flow of a program. They are thrown at runtime and can be caught and handled using try-catch blocks.
46. What are checked exceptions and unchecked exceptions?
- Checked exceptions are checked at compile-time, meaning the compiler ensures they are properly handled (either by catching them or declaring them to be thrown).
- Unchecked exceptions (RuntimeExceptions) are not checked at compile-time.
47. What is the purpose of the `finally` block in exception handling?
- The `finally` block is used to execute important code such as closing resources (like database connections or file streams), whether an exception is thrown or not.
48. Can you have multiple catch blocks for a single try block?
- Yes, a single try block can have multiple catch blocks to handle different types of exceptions.
49. What is the difference between `throw` and `throws` in Java?
- `throw` is used to explicitly throw an exception from a method or block of code.
- `throws` is used in method signatures to declare that the method may throw certain exceptions.
### 10. Multithreading
50. What is multithreading in Java?
- Multithreading refers to the ability of a program to execute multiple threads simultaneously, allowing for concurrent execution of tasks.
51. How do you create a thread in Java?
- You can create a thread by extending the `Thread` class or implementing the `Runnable` interface. For example:
```java
class MyThread extends Thread {
public void run() {
// Thread execution logic
}
}
// Create and start the thread
MyThread thread = new MyThread();
thread.start();
```
52. What is the difference between `Thread` class and `Runnable` interface?
- `Thread` class is a class that represents a thread of execution, whereas `Runnable` interface is a functional interface representing a task that can be executed by a thread.
53. How do you synchronize threads in Java?
- Threads can be synchronized using the `synchronized` keyword, which can be applied to methods or blocks of code. This ensures that only one thread can access the synchronized code at a time.
54. What is deadlock in multithreading?
- Deadlock occurs when two or more threads are blocked forever, waiting for each other to release resources.
55. What is the `volatile` keyword in Java?
- The `volatile` keyword in Java is used to indicate that a variable's value will be modified by multiple threads.
### 11. Collections Framework
56. What is the Collections Framework in Java?
- The Collections Framework is a unified architecture for representing and manipulating collections (lists, sets, maps, etc.) in Java.
57. What is the difference between `List`, `Set`, and `Map` in Java?
- `List` is an ordered collection that allows duplicate elements.
- `Set` is a collection that does not allow duplicate elements.
- `Map` is a collection that maps keys to values and does not allow duplicate keys.
58. What is an `ArrayList` in Java?
- `ArrayList` is a resizable array implementation of the `List` interface in Java. It allows dynamic resizing of the underlying array.
59. How do you iterate through a `List` in Java?
- You can iterate through a `List` using a `for-each` loop or using the `Iterator` interface. For example:
```java
List<String> list = new ArrayList<>();
list.add("A");
list.add("B");
for (String item : list) {
System.out.println(item);
}
```
60. What is the difference between `ArrayList` and `LinkedList`?
- `ArrayList` uses a dynamic array for storing elements and is good for random access and traversal.
- `LinkedList` uses a doubly linked list and is good for frequent insertions and deletions.
### 12. Generics
61. What are generics in Java?
- Generics allow classes and methods to operate on objects of various types while providing compile-time type safety.
62. Why are generics used in Java?
- Generics provide type safety by allowing you to specify the type of objects that a collection or class can contain or operate on.
63. What is the difference between a raw type and a generic type?
- A raw type is a non-parameterized type (e.g., `ArrayList`) whereas a generic type is parameterized with type information (e.g., `ArrayList<String>`).
64. How do you define a generic class in Java?
- You can define a generic class by using angle brackets `<>` and specifying one or more type parameters. For example:
```java
public class MyGenericClass<T> {
private T value;
public void setValue(T value) {
this.value = value;
}
public T getValue() {
return value;
}
}
```
65. What is a wildcard in generics?
- Wildcards (`?`) in generics allow flexibility in specifying generic types. `? extends T` means any subtype of `T`, and `? super T` means any supertype of `T`.
### 13. Lambda Expressions and Functional Interfaces
66. What are lambda expressions in Java?
- Lambda expressions introduce functional programming concepts to Java, allowing you to concisely express instances of single-method interfaces (functional interfaces).
67. What is a functional interface in Java?
- A functional interface is an interface that contains exactly one abstract method. It can have any number of default methods or static methods.
68. How do you use lambda expressions in Java?
- Lambda expressions are typically used to implement functional interfaces. For example:
```java
// Using lambda expression to implement a functional interface
MyFunctionalInterface func = () -> {
System.out.println("Hello, lambda!");
};
func.myMethod();
```
69. What is the `@FunctionalInterface` annotation?
- `@FunctionalInterface` annotation is used to ensure that an interface qualifies as a functional interface. It is optional but recommended for clarity.
### 14. Stream API
70. What is the Stream API in Java?
- Stream API is a new abstraction in Java 8 that allows you to process collections of objects in a functional manner.
71. What are the characteristics of Stream API?
- Stream API is declarative (functional style), supports parallel processing, and does not modify the underlying data structure.
72. How do you create a Stream in Java?
- You can create a Stream from a collection using the `stream()` method or from an array using `Arrays.stream()`.
73. What is the difference between intermediate and terminal operations in Stream API?
- Intermediate operations return a new Stream and allow chaining (e.g., `filter()`, `map()`).
- Terminal operations produce a result or side-effect and are the last operation in a Stream pipeline (e.g., `forEach()`, `collect()`).
74. How do you iterate through a Stream in Java?
- You can iterate through a Stream using terminal operations like `forEach()` or by converting it back to a collection using `collect()`.
### 15. File Handling
75. How do you read from a file in Java?
- Files can be read in Java using classes from the `java.io` package such as `File`, `FileReader`, `BufferedReader`, etc.
76. How do you write to a file in Java?
- Files can be written in Java using classes from the `java.io` package such as `File`, `FileWriter`, `BufferedWriter`, etc.
77. What is the difference between `File` and `FileInputStream`/`FileOutputStream` in Java?
- `File` class represents the actual file or directory on disk, whereas `FileInputStream` and `FileOutputStream` classes are used to read from and write to files, respectively.
### 16. Annotations
78. What are annotations in Java?
- Annotations provide metadata (data about data) about classes, methods, fields, etc., which can be processed at compile-time or runtime.
79. What is the `@Override` annotation used for?
- `@Override` annotation is used to indicate that a method in a subclass is overriding a method in its superclass.
80. What is the `@SuppressWarnings` annotation used for?
- `@SuppressWarnings` annotation is used to suppress compiler warnings for a specific part of code.
### 17. Java Virtual Machine (JVM)
81. What is the JVM (Java Virtual Machine)?
- JVM is an abstract machine that provides a runtime environment in which Java bytecode can be executed.
82. What are the JVM languages?
- JVM supports languages other than Java, such as Kotlin, Scala, Gro
ovy, etc., as long as they compile to Java bytecode.
83. What is JIT (Just-In-Time) compiler in Java?
- JIT compiler is a part of JVM that improves the performance of Java applications by compiling bytecode into native machine code at runtime.
### 18. Java Memory Management
84. How does Java handle memory management?
- Java uses automatic memory management (garbage collection) to manage memory allocation and deallocation.
85. What is garbage collection in Java?
- Garbage collection is the process of automatically reclaiming unused memory (objects that are no longer referenced) in Java.
86. What are the different types of memory in Java?
- Java memory consists of stack memory (for method calls and local variables) and heap memory (for objects and instances).
87. What is the `finalize()` method in Java?
- `finalize()` method is a protected method in `Object` class that can be overridden to perform cleanup operations on an object before it is garbage collected.
### 19. Serialization and Deserialization
88. What is serialization in Java?
- Serialization is the process of converting an object into a byte stream so that it can be saved to a file or sent over a network.
89. What is deserialization in Java?
- Deserialization is the process of converting a byte stream back into an object so that it can be used in a Java application.
90. How do you make a class serializable in Java?
- To make a class serializable, implement the `Serializable` interface. For example:
```java
import java.io.Serializable;
public class MyClass implements Serializable {
// Class definition
}
```
### 20. JDBC (Java Database Connectivity)
91. What is JDBC in Java?
- JDBC is an API that allows Java applications to interact with databases.
92. What are the steps to connect to a database using JDBC?
- Steps include loading the JDBC driver, establishing a connection, creating a statement, executing SQL queries, processing results, and closing the connection.
93. What is a `PreparedStatement` in JDBC?
- `PreparedStatement` is a precompiled SQL statement that allows execution of parameterized queries. It helps prevent SQL injection attacks and improves performance.
94. What is connection pooling in JDBC?
- Connection pooling is a technique used to manage a pool of database connections that can be reused, thus reducing the overhead of creating new connections.
### 21. Networking in Java
95. What is networking in Java?
- Networking in Java involves communicating between computers over a network using classes from the `java.net` package.
96. How do you create a `Socket` in Java?
- You can create a `Socket` object to establish a connection to a server using its IP address and port number. For example:
```java
Socket socket = new Socket("127.0.0.1", 8080);
```
97. What is a `ServerSocket` in Java?
- `ServerSocket` is used by servers to listen for incoming client connections on a specified port.
98. What is the difference between TCP and UDP in Java networking?
- TCP (Transmission Control Protocol) provides reliable, connection-oriented communication, whereas UDP (User Datagram Protocol) provides connectionless communication that is faster but less reliable.
### 22. Reflection in Java
99. What is reflection in Java?
- Reflection in Java allows examining or modifying the runtime behavior of applications.
100. How do you get the class object of a class in Java?
- You can get the class object of a class by using `.class` syntax or by calling `.getClass()` method on an object.
101. What are some use cases of reflection in Java?
- Use cases include examining annotations, inspecting classes, invoking methods dynamically, and accessing private fields/methods.
### 23. Java Annotations
102. What are Java annotations used for?
- Annotations provide metadata about classes, methods, fields, etc., which can be processed at compile-time or runtime.
103. What is `@Override` annotation used for?
- `@Override` annotation indicates that a method in a subclass is overriding a method in its superclass.
104. What is `@SuppressWarnings` annotation used for?
- `@SuppressWarnings` annotation is used to suppress compiler warnings for a specific part of code.
### 24. Java Design Patterns
105. What are design patterns in Java?
- Design patterns are proven solutions to common problems encountered in software design.
106. What are some common design patterns in Java?
- Examples include Singleton, Factory, Observer, Strategy, Builder, Adapter, and Decorator patterns.
107. Explain the Singleton design pattern in Java.
- Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance.
### 25. Java 8 Features
108. What are the new features introduced in Java 8?
- Java 8 introduced lambda expressions, Stream API, default methods in interfaces, method references, and functional interfaces.
109. What are lambda expressions in Java 8?
- Lambda expressions allow you to treat functionality as a method argument, or to create concise classes that implement single-method interfaces.
110. How do you use the Stream API in Java 8?
- Stream API allows processing collections of objects in a functional manner, with support for operations like `map()`, `filter()`, `reduce()`, etc.
### 26. Java 9, 10, 11 Features
111. What are some new features introduced in Java 9?
- Java 9 introduced modules (Project Jigsaw), `jshell` (an interactive REPL), and improvements to the Stream API.
112. What are some new features introduced in Java 10 and 11?
- Java 10 introduced local-variable type inference (`var` keyword), while Java 11 introduced HTTP Client API, `var` for lambda parameters, and improvements to the `String` class.
### 27. Java Best Practices
113. What are some best practices for writing Java code?
- Use meaningful variable and method names, follow naming conventions, write modular and maintainable code, handle exceptions properly, and use version control.
114. Why should you use immutable classes in Java?
- Immutable classes provide several benefits including thread safety, simplicity, and no side effects, making them easier to reason about and use in concurrent environments.
115. What is the importance of exception handling in Java?
- Exception handling improves robustness by allowing you to gracefully handle errors and exceptions that may occur during program execution.
### 28. Java Coding Exercises
116. Can you give an example of a simple Java coding exercise?
- Sure! Here's a simple exercise to find the factorial of a number:
```java
public class FactorialExample {
public static void main(String[] args) {
int number = 5;
long factorial = 1;
for (int i = 1; i <= number; ++i) {
factorial *= i;
}
System.out.printf("Factorial of %d = %d", number, factorial);
}
}
```
117. What are some resources for practicing Java coding exercises?
- Websites like LeetCode, HackerRank, and Codeforces offer a wide range of Java coding challenges and exercises for practice.
### 29. Java Interview Preparation Tips
118. How should you prepare for a Java interview?
- Review core Java concepts, practice coding exercises, understand common algorithms and data structures, and be prepared to discuss your projects and experiences.
119. What are some common Java interview questions for freshers?
- Questions may cover topics like OOP concepts, collections, exception handling, multithreading, and basic algorithms.
### 30. Miscellaneous
120. What are the differences between `StringBuilder` and `StringBuffer`?
- Both `StringBuilder` and `StringBuffer` are used to create mutable string objects, but `StringBuffer` is synchronized (thread-safe) whereas `StringBuilder` is not.
121. What is the `transient` keyword in Java?
- `transient` keyword is used to indicate that a member variable should not be serialized when the object is serialized.
122. What is the `volatile` keyword in Java?
- `volatile` keyword is used to indicate that a variable's value will be modified by multiple threads.
123. What are some differences between `HashMap` and `Hashtable`?
- `HashMap` is not synchronized (not thread-safe) whereas `Hashtable` is synchronized (thread-safe).
- `HashMap` allows `null` values and one `null` key whereas `Hashtable` does not allow `null` values or keys.
124. Explain the `finalize()` method in Java.
- `finalize()` method is called by the garbage collector before an object is garbage collected. It can be overridden to perform cleanup operations on the object.
These questions cover a wide range of topics and should help you prepare thoroughly for a Java interview as a fresher. Make sure to understand the concepts and practice coding examples to strengthen your understanding further.
TheTechStudioz©2023
Reframe your inbox
Subscribe to our Blog and never miss a story.
We care about your data in our privacy policy.